First of all you need to create a new project, Here I am using Visual Studio 2008
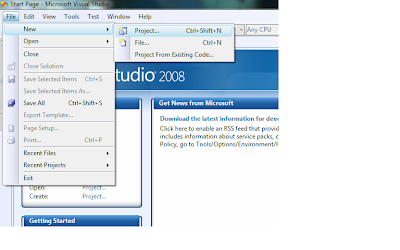
-- Windows Forms Application
Now we will see something like this Called form
Third Step is to Design the Form
--- First Select button from Toolbar
--- Second Select List View from Toolbar
---Select View as you need, Here I select Detail
After that Click on ADD and edit text as you want to present on header of ListView
Here all the Setting is complete Next Task is to CODE the cs File
Here is the Code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlServerCe;
namespace List_view
{
public partial class Form1 : Form
{
public SqlCeConnection con = new SqlCeConnection(@"Connection string ");
public Form1()
{
InitializeComponent();
}
private void Form1_Shown(object sender, EventArgs e)
{
try
{
con.Open();
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}
private void PopulateList_Click(object sender, EventArgs e)
{
listView1.Items.Clear();
SqlCeCommand cmd2 = new SqlCeCommand("Select * from Login", con);
SqlCeDataAdapter da = new SqlCeDataAdapter(cmd2);
try
{
DataTable table = new DataTable();
da.Fill(table);
foreach (DataRow dr in table.Rows)
{
ListViewItem item = new ListViewItem(dr["id"].ToString());
item.SubItems.Add(dr["password"].ToString());
listView1.Items.Add(item);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}
private void ClearList_Click(object sender, EventArgs e)
{
listView1.Items.Clear();
}
}
}